Welcome to a tutorial on how to add new rows to a CSV file in PHP. So you need to append, prepend, or insert a new row into an existing CSV file? Well, we can append a new row to a CSV file in PHP using only 3 lines of code:
$csv = fopen("demo.csv", "a");
fputcsv($csv, ["A", "B"]);
fclose($csv);
That’s all, but how about prepending and inserting at an exact location? Read on for more examples!
TABLE OF CONTENTS
DOWNLOAD & NOTES
Here is the download link to the example code, so you don’t have to copy-paste everything.
EXAMPLE CODE DOWNLOAD
The example code is released under the MIT license, so feel free to build on top of it or use it in your own project.
SORRY FOR THE ADS...
But someone has to pay the bills, and sponsors are paying for it. I insist on not turning Code Boxx into a "paid scripts" business, and I don't "block people with Adblock". Every little bit of support helps.
Buy Me A Coffee Code Boxx eBooks
PHP ADD NEW ROW TO CSV
All right, let us now get into the examples of adding new rows into an existing CSV file in PHP.
TUTORIAL VIDEO
1) APPENDING ROWS TO CSV FILE
<?php
// (A) OPEN CSV FILE
$csv = fopen("demo.csv", "a");
// (B) APPEND TO CSV FILE
fputcsv($csv, ["A", "B"]);
foreach ([
["C", "D"],
["E", "F"]
] as $row) { fputcsv($csv, $row); }
// (C) CLOSE CSV FILE
fclose($csv);
As in the introduction above, appending new rows to a CSV is an easy 3 steps – fopen()
, fputcsv()
, and fclose()
.
2) PREPENDING ROWS TO CSV FILE
<?php
// (A) READ EXISTING CSV FILE INTO ARRAY
$csv = fopen("demo.csv", "r");
$rows = [];
while (($r=fgetcsv($csv)) !== false) { $rows[] = $r; }
fclose($csv);
// (B) PREPEND NEW ROWS
$rows = array_merge([
["G", "H"],
["I", "J"]
], $rows);
// (C) SAVE UPDATED CSV
$csv = fopen("demo.csv", "w");
foreach ($rows as $r) { fputcsv($csv, $r); }
fclose($csv);
Appending new rows is easy. But sadly, there are no ways to insert rows to the top of the CSV file directly.
- We read the entire CSV file into an array first.
- Using
array_merge()
, we prepend new rows to the existing data. - Finally, write the updated rows back into the CSV file.
Roundabout, but it works.
3) INSERTING ROWS INTO CSV FILE
<?php
// (A) READ EXISTING CSV FILE INTO ARRAY
$csv = fopen("demo.csv", "r");
$rows = [];
while (($r=fgetcsv($csv)) !== false) { $rows[] = $r; }
fclose($csv);
// (B) PREPEND NEW ROWS
array_splice($rows, 4, 0, [["K", "L"]]);
// (C) SAVE UPDATED CSV
$csv = fopen("demo.csv", "w");
foreach ($rows as $r) { fputcsv($csv, $r); }
fclose($csv);
This is a “follow-up” of the above example – If you want to insert at an exact location, use array_splice()
instead of array_merge()
.
4) SEARCH & INSERT ROW INTO CSV FILE
<?php
// (A) READ EXISTING CSV FILE INTO ARRAY
$csv = fopen("demo.csv", "r");
$rows = [];
while (($r=fgetcsv($csv)) !== false) { $rows[] = $r; }
fclose($csv);
// (B) INSERT BEFORE "JON DOE"
$at = 0;
foreach ($rows as $i=>$r) {
if (in_array("Jon Doe", $r)) { $at = $i; break; }
}
array_splice($rows, $at, 0, [["M", "N"]]);
// (C) SAVE UPDATED CSV
$csv = fopen("demo.csv", "w");
foreach ($rows as $r) { fputcsv($csv, $r); }
fclose($csv);
The previous example works, only if you know exactly where to insert. If you don’t know the exact row, the only way is to loop all the entries and search for where to insert.
EXTRAS
That’s all for the tutorial, and here is a small section on some extras and links that may be useful to you.
TAKE NOTE – LIMITED SYSTEM RESOURCES
The above examples for prepend and insert will read the entire CSV file into memory. While most modern servers can handle big CSV files without any issues, there is still something called “limited system resources”. If you are dealing with massive CSV files, it will be wise to split them or create a database altogether.
INFOGRAPHIC CHEAT SHEET
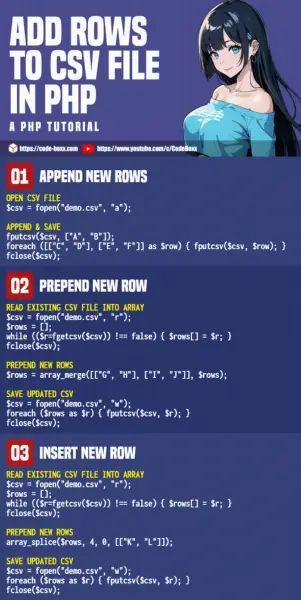
LINKS & REFERENCES
- PHP – fgetcsv | fputcsv
- Display CSV In HTML Table – Code Boxx
THE END
Thank you for reading, and we have come to the end. I hope that it has helped you to better understand, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!