Welcome to a quick tutorial on how to create a non-editable text field in HTML and Javascript. Want to “fix” a text field in HTML or Javascript? Prevent the user from changing the value?
- To create a non-editable text field in HTML, we can either attach the
readonly
ordisabled
attribute.<input type="text" readonly>
<input type="text" disabled>
- We can also set the
readonly
ordisabled
attribute in Javascript to create non-editable text fields.document.getElementById("ID").readOnly = true;
document.getElementById("ID").disabled = true;
That covers the quick basics, but read on for more detailed examples!
ⓘ I have included a zip file with all the source code at the start of this tutorial, so you don’t have to copy-paste everything… Or if you just want to dive straight in.
TLDR – QUICK SLIDES
[web_stories_embed url=”https://code-boxx.com/web-stories/non-editable-html-text-field/” title=”Non-Editable HTML Text Field” poster=”https://code-boxx.com/wp-content/uploads/2022/03/STORY-HTML-20230505.webp” width=”360″ height=”600″ align=”center”]
Fullscreen Mode – Click Here
TABLE OF CONTENTS
DOWNLOAD & NOTES
Firstly, here is the download link to the example code as promised.
QUICK NOTES
If you spot a bug, feel free to comment below. I try to answer short questions too, but it is one person versus the entire world… If you need answers urgently, please check out my list of websites to get help with programming.
EXAMPLE CODE DOWNLOAD
Click here to download all the example source code, I have released it under the MIT license, so feel free to build on top of it or use it in your own project.
NON-EDITABLE TEXT FIELDS
All right, let us now get into the examples of non-editable text fields in HTML.
EXAMPLE 1) HTML READ-ONLY & DISABLED
<!-- (A) READONLY -->
<input type="text" value="Hello" readonly>
<!-- (B) DISABLED -->
<input type="text" value="World" disabled>
<!-- (C) ALSO WORKS ON TEXTAREA -->
<textarea readonly>Hello</textarea>
<textarea disabled>World</textarea>
Yep, it’s that simple. As in the introduction, just attach a readonly
or disabled
property to the text field. What is the difference between the two? Semantically speaking:
readonly
fields are still “valid”. It’s just that the value is fixed and cannot be changed.disabled
fields are “invalid”. They may no longer apply because of another choice in the form. For example, choosing “I have not used the product before” and disabling a “product feedback” text field.
EXAMPLE 2) JAVASCRIPT SET READ-ONLY & DISABLED
<script>
/* (A) ENABLE TEXT FIELD */
function enable () {
var demo = document.getElementById("demo");
demo.value = "Enabled";
demo.disabled = false;
//demo.readOnly = false;
}
/* (B) DISABLE TEXT FIELD */
function disable () {
var demo = document.getElementById("demo");
demo.value = "Disabled";
demo.disabled = true;
//demo.readOnly = true;
}
</script>
<input type="text" id="demo">
<input type="button" value="Enable" onclick="enable()">
<input type="button" value="Disable" onclick="disable()">
Lastly, here’s a quick example to showcase how Javascript can also set/unset the readonly
and disabled
properties – This should come in handy for you guys who are working on dynamic forms.
EXAMPLE 3) STYLING NON-EDITABLE TEXT FIELDS IN CSS
<style>
/* (A) READONLY */
input:read-only, textarea:read-only {
color: #296aad;
background: #f7f6ff;
border: 1px solid #ddd;
}
/* (B) DISABLED */
input:disabled, textarea:disabled {
color: #9e9e9e;
background: #efefef;
border: 1px solid #ddd;
}
</style>
<input type="text" value="Hello" readonly>
<input type="text" value="World" disabled>
<textarea readonly>Hello</textarea>
<textarea disabled>World</textarea>
For you guys who want to customize your own non-editable fields, simply use the :read-only
or :disabled
pseudo-class in CSS.
EXTRA BITS & LINKS
That’s all for the tutorial, and here is a small section on some extras and links that may be useful to you.
LINKS & REFERENCES
- HTML attribute: readonly – MDN
- HTML attribute: disabled – MDN
- :read-only – MDN
- :disabled – MDN
INFOGRAPHIC CHEAT SHEET
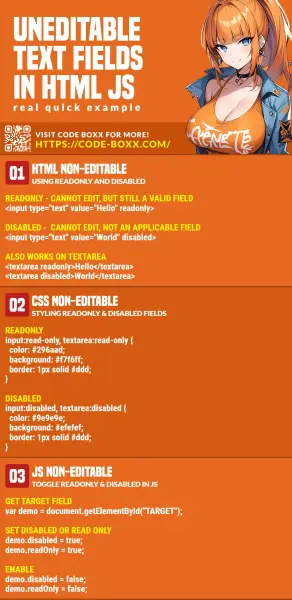
THE END
Thank you for reading, and we have come to the end. I hope that it has helped you to better understand, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!