Welcome to a tutorial on how to abort Javascript execution. Need to manually stop a piece of script for some reason?
Client-side Javascript does not have a native abort function, but there are various alternatives to abort Javascript execution:
- In a function, simply
return false
orundefined
. - Manually
throw new Error("ERROR")
in a function. - Set a function to run on a timer –
var timer = setInterval(FUNCTION, 1000)
. Then clear it to stop –clearInterval(timer)
- Run the script with workers that can be terminated.
- Use
window.stop()
to prevent the page from loading and running. - For NodeJS only – Use
process.abort()
orprocess.exit()
.
But just how does each method work? Let us walk through some examples in this guide. Read on to find out!
TLDR – QUICK SLIDES
[web_stories_embed url=”https://code-boxx.com/web-stories/how-to-abort-javascript-execution/” title=”How To Abort Javascript Execution” poster=”https://code-boxx.com/wp-content/uploads/2021/11/STORY-JS-20230518.webp” width=”360″ height=”600″ align=”center”]
Fullscreen Mode – Click Here
TABLE OF CONTENTS
ABORT JAVASCRIPT EXECUTION
All right, let us now go through all the possible methods to stop or abort Javascript execution.
METHOD 1) RETURN FALSE
function test () {
// (A) DO YOUR STUFF AS USUAL
console.log("Test started");
let i = 0;
i++;
// (B) ABORT - RETURN "UNDEFINED"
if (i != 0) { return; }
// (C) RETURN RESULT IF OK
console.log("This part will not run");
return i;
}
// (D) FIRE TEST()
let i = test();
console.log(i);
// (E) BEST TO HANDLE THE ABORTION GRACEFULLY
if (i==undefined) {
// HANDLE ABORT
}
This is one of the most graceful and “non-hacker” ways that I will recommend using – Just return false
or return undefined
in the function. It should be very straightforward and easy to understand, even for beginners.
P.S. You might also want to handle what happens after the abortion. Maybe show an “error” message or something.
METHOD 2) THROW EXCEPTION
function test () {
// (A) DO YOUR STUFF AS USUAL
console.log("Test started");
let i = 0;
i++;
// (B) ABORT - THROW ERROR
if (i != 0) {
throw new Error("Manual Abort Script");
}
// (C) RETURN RESULT IF OK
console.log("This part will not run");
return i;
}
// (D) BEST TO TRY-CATCH FOR A GRACEFUL SCRIPT
try {
let x = test();
} catch (err) {
console.log(err);
}
Moving on, this is a “popular” method that I found on the Internet. Yep, it is funky to throw an “error” when it is not actually an error… But this is a guaranteed way to abort and stop scripts from proceeding any further. To not “crash” the script altogether, it is recommended to use a try-catch
block to handle things gracefully – Maybe show the user a “script aborted” notification.
P.S. I will still recommend using the above return;
or return false;
instead – It is “softer” and “logically correct”.
METHOD 3) TIMER STOP
// (A) SET TIMER & RUN
var timer = setInterval(
() => console.log("Running"), 3000
);
// (B) "FORCE" ABORT IMMEDIATELY
clearInterval(timer);
// (C) OR SET A "TIMEOUT"
setTimeout(() => {
clearInterval(timer);
console.log("Stopped");
}, 1000);
This is yet another one that I found on the Internet. The whole idea of this method is to put the script on a timer, and simply call clearInterval()
to abort it. While it works, it is also kind of useless unless you have a script that runs in a loop or timer…
METHOD 4) WORKER TERMINATE
4A) WHAT ARE WORKERS?
For you beginner code ninjas who have not heard of workers – This is basically the Javascript way to do multithreading, allowing scripts to run in the background, allowing multiple scripts to run in parallel. If you want to learn more, I will leave a link to my other worker tutorial in the extras section below.
4B) THE MAIN SCRIPT
// (A) CREATE NEW WORKER
var theWorker = new Worker("4b-worker.js");
// (B) WHEN THE WORKER IS DONE
theWorker.onmessage = evt => {
console.log("Worker script has completed.");
console.log(evt.data);
};
// (C) SEND DUMMY DATA TO WORKER
// ! WORKER SCRIPT CANNOT ACCESS DOM DIRECTLY !
theWorker.postMessage({
Name : "John Doe",
Email : "john@doe.com"
});
// (D) ABORT AND TERMINATE WORKER
// theWorker.terminate();
THE WORKER SCRIPT
// (A) START ONLY WHEN DATA RECEIVED
onmessage = evt => {
// (B) DATA RECEIVED
console.log("Worker has received data and started.");
console.log(evt.data);
// (C) DO PROCESSING HERE
// ...
// (D) REPLY RESULT
postMessage({
status: 0,
message: "OK"
});
};
THE EXPLANATION
Workers are kind of confusing at first look, but it really just works somewhat like an AJAX call – There are 2 parts to it, the main script 4a-main.js
and the worker script 4b-worker.js
.
- First, we start by creating a new worker
var theWorker = new Worker("4b-worker.js")
in the main script. - Take extra note that worker scripts have no access to the DOM tree. I.E.
document.getElementByXX()
will not work in4b-worker.js
. - This is why we have to send data to the worker in the main script –
theWorker.postMessage( ... );
- Next in the worker script, we begin processing only when data is received –
onmessage = function (evt) { ... }
. - When the worker is done, it returns a message back to the main script –
postMessage( ... )
. - Finally, back in the main script, we handle when the worker is done –
theWorker.onmessage = function (evt) { ... }
.
As for the best part, we can abort the worker at any point in time using theWorker.terminate()
.
METHOD 5) WINDOW STOP
<script src="5a-stop.js"></script>
<script src="5b-stop.js"></script>
// (A) DO YOUR STUFF
var first = 123;
var second = 456;
var third = first + second;
console.log(third);
// (B) STOP LOADING
window.stop();
console.log("This will still run...");
console.log("THIS WILL NOT RUN");
Just use the window.stop()
to stop the rest of the page from loading… Yep, it kind of works, and will stop all other scripts from loading/running. But as you might have guessed, this will also stop the HTML page from fully loading. 😐 Not really useful, unless you want to do an “emergency brake”.
METHOD 6) NODEJS ABORT & EXIT
// THIS IS FOR NODEJS ONLY
// (A) DO YOUR STUFF
var first = 123;
var second = 456;
var third = first + second;
console.log(third);
// (B) ABORT OR EXIT
// ABORT = IMMEDIATE (ROUGH)
// EXIT = AS QUICKLY AS POSSIBLE (GRACEFUL)
// process.abort();
process.exit();
console.log("THIS WILL NOT RUN");
This is for NodeJS only, use the process.abort()
and process.exit()
functions to stop the execution. The difference between them is that abort()
will stop immediately, and exit()
will stop as soon as possible… That is, exit()
is more graceful with the cleaning up, and not an abrupt “suddenly stop everything”.
DOWNLOAD & NOTES
Here is the download link to the example code, so you don’t have to copy-paste everything.
SORRY FOR THE ADS...
But someone has to pay the bills, and sponsors are paying for it. I insist on not turning Code Boxx into a "paid scripts" business, and I don't "block people with Adblock". Every little bit of support helps.
Buy Me A Coffee Code Boxx eBooks
EXAMPLE CODE DOWNLOAD
Click here for the source code on GitHub gist, just click on “download zip” or do a git clone. I have released it under the MIT license, so feel free to build on top of it or use it in your own project.
EXTRA BITS & LINKS
That’s all for this guide, and here is a small section on some extras and links that may be useful to you.
LINKS & REFERENCES
- How to terminate the script in JavaScript? – Stack Overflow
- How to stop the execution of a function with JavaScript? – Tutorials Point
- Javascript Multithreading – Workers For Beginners – Code Boxx
- Worker Terminate – MDN
- Error – MDN
- process.abort() | process.exit() – NodeJS
- Window.stop() – MDN
TUTORIAL VIDEO
INFOGRAPHIC CHEAT SHEET
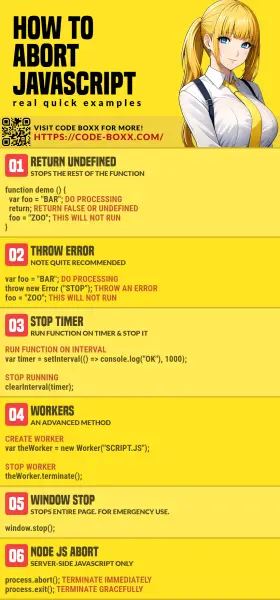
THE END
Thank you for reading, and we have come to the end of this guide. I hope that it has helped you with your project, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!
Thanks, the info about throwing an Error() was what I needed. I was trying to terminate execution inside a file that gets minimized before it ends up in a full file; but a return statement without appearing to be in a function was making the minimizer get confused. Error() seems to be the solution. Cheers