Welcome to a quick tutorial and examples of how to toggle fullscreen mode with Javascript. Yes, the old grandmother’s age of the Internet is over now. Modern web browsers can be switched to full-screen mode in Javascript easily.
- To enter fullscreen mode for the entire page –
document.documentElement.requestFullscreen()
- To enter fullscreen mode for a specific element –
document.getElementById("ID").requestFullscreen()
- Lastly, use
document.exitFullscreen()
to exit fullscreen.
Yep, that’s all the basics. But read on if you need an actual example!
TLDR – QUICK SLIDES
[web_stories_embed url=”https://code-boxx.com/web-stories/toggle-fullscreen-in-javascript/” title=”Toggle Fullscreen In Javascript” poster=”https://code-boxx.com/wp-content/uploads/2021/11/STORY-JS-20230518.webp” width=”360″ height=”600″ align=”center”]
Fullscreen Mode – Click Here
TABLE OF CONTENTS
JAVASCRIPT FULLSCREEN MODE
All right, let us now get into the example of toggling fullscreen mode in Javascript.
FULLSCREEN DEMO
PART 1) TOGGLE FULLSCREEN MODE
<!-- (A) IMAGE FOR TESTING FULLSCREEN -->
<img id="demo" src="chick.jpg">
<!-- (B) FULLSCREEN BUTTONS -->
<div>
<!-- (B1) ENTIRE PAGE -->
<input type="button" value="Entire Page"
onclick="document.documentElement.requestFullscreen()">
<!-- (B2) IMAGE ONLY -->
<input type="button" value="Image Only"
onclick="document.getElementById('demo').requestFullscreen()">
<!-- (B3) EXIT FULLSCREEN -->
<input type="button" value="Exit"
onclick="document.exitFullscreen();">
</div>
Yep, this is as advertised in the introduction snippet.
- Use
requestFullscreen()
to enter the fullscreen mode - Use
exitFullscreen()
to exit full screen.
It’s really that simple.
document.documentElement
refers to the entire HTML document. Sodocument.documentElement.requestFullscreen()
will set the entire page into fullscreen mode.document.getElementById(TARGET).requestFullscreen()
is self-explanatory… We only set that particular section of the page into fullscreen mode.
PART 2) DETECTING FULLSCREEN CHANGE
<!-- (A) FULLSCREEN BUTTONS -->
<input type="button" value="Fullscreen"
onclick="document.documentElement.requestFullscreen()">
<input type="button" value="Exit"
onclick="document.exitFullscreen();">
<!-- (B) JAVASCRIPT -->
<script>
// (B) LISTEN TO FULLSCREEN TOGGLE
document.addEventListener("fullscreenchange", () => {
if (document.fullscreenElement===null) {
console.log("Exited fullscreen");
} else {
console.log("Entered fullscreen");
}
});
// (C) ON FULLSCREEN ERROR
document.addEventListener("fullscreenerror", evt => console.error(evt));
</script>
For you guys who want more controls, to do some things when the user toggles fullscreen:
- The
fullscreenchange
event is triggered whenever fullscreen mode is toggled. document.fullscreenElement
contains the current fullscreen element. If this isnull
, the user is not in fullscreen mode.- The
fullscreenerror
event is triggered when the user has trouble entering fullscreen mode.
EXTRA) FULLSCREEN ONLY CSS
#demo:fullscreen { background: white }
For those who are interested, notice that single line of CSS. Yep, we can use :fullscreen
to apply styles on elements only when they are in fullscreen mode.
DOWNLOAD & NOTES
Here is the download link to the example code, so you don’t have to copy-paste everything.
SORRY FOR THE ADS...
But someone has to pay the bills, and sponsors are paying for it. I insist on not turning Code Boxx into a "paid scripts" business, and I don't "block people with Adblock". Every little bit of support helps.
Buy Me A Coffee Code Boxx eBooks
EXAMPLE CODE DOWNLOAD
Click here for the source code on GitHub gist, just click on “download zip” or do a git clone. I have released it under the MIT license, so feel free to build on top of it or use it in your own project.
EXTRA BITS & LINKS
That’s all for the main tutorial, and here is a small section on some extras and links that may be useful to you.
COMPATIBILITY CHECKS
- Fullscreen API – CanIUse
- Arrow Functions – CanIUse
I will recommend doing feature detection and using a Polyfill – Check out Modernizr.
LINKS & REFERENCES
- Fullscreen API – MDN
- Enhance fullscreen on mobile devices, lock screen orientation – Code Boxx
INFOGRAPHIC CHEAT SHEET
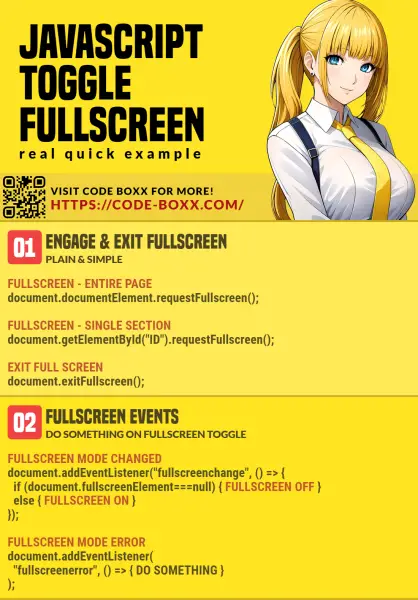
THE END
Thank you for reading, and we have come to the end of this guide. I hope that it has helped you with your project, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!
I implemented full screen mode based on the examples above. However, if I move to another page on the website, the fullscreen mode is disabled.
How can I permanently enable it regardless of what page I got to on a website? Just like the native full screen CMD+CTRL+F or F11 in Windows. It’s always full screen regardless of the page in the website I visit.
You have somewhat answered your own question – This is a browser behavior and cannot be overridden.
https://developer.mozilla.org/en-US/docs/Web/API/Fullscreen_API => Navigating to another page, changing tabs, or switching to another application using any application switcher (or Alt-Tab) will likewise exit full-screen mode.