Welcome to a tutorial and example on how to send a Javascript fetch request with GET query parameters. New to the fetch API, and trying to pass in some query parameters?
To send GET query parameters with Javascript fetch, simply create a new URL object and append the search parameters:
var data = { "KEY" : "VALUE" };
var url = new URL("http://site.com/API");
for (let k in data) { url.searchParams.append(k, data[k]); }
fetch(url);
That covers the quick basics, but read on for detailed examples.
TLDR – QUICK SLIDES
[web_stories_embed url=”https://code-boxx.com/web-stories/send-get-params-with-javascript-fetch/” title=”Send GET Params With Javascript Fetch” poster=”https://code-boxx.com/wp-content/uploads/2021/11/STORY-JS-20230518.webp” width=”360″ height=”600″ align=”center”]
Fullscreen Mode – Click Here
TABLE OF CONTENTS
JAVASCRIPT FETCH WITH GET PARAMS
All right, let us now get into the examples of Javascript fetch with GET parameters.
EXAMPLE 1) APPEND SEARCH PARAMS
<script>
function fetchGet () {
// (A) DATA TO SEND
var data = {
name : "jon doe",
email : "jon@doe.com"
};
// (B) BUILD URL
var url = new URL("http://site.com/0-dummy.php");
for (let k in data) { url.searchParams.append(k, data[k]); }
console.log(url);
// (C) FETCH WITH GET SEARCH PARAMS
fetch(url)
// (D) RETURN SERVER RESPONSE AS TEXT
.then(res => {
console.log(res);
if (res.status != 200) { throw new Error("Bad Server Response"); }
return res.text();
})
.then(res => console.log(res))
// (E) HANDLE ERRORS (OPTIONAL)
.catch(err => console.error(err));
}
</script>
<input type="button" value="Fetch Get" onclick="fetchGet()">
This is the “full version” of the introduction snippet. As you can see, the parameters are attached to the URL itself, it has nothing much to do with fetch()
. Take note of how we generate the URL though:
var url = new URL();
url.searchParams.append(KEY, VALUE);
The URL search params API is well-supported in all modern browsers at the time of writing, but take note that it will not work on the old Internet Exploders (caniuse.com).
EXAMPLE 2) MANUAL URL ENCODE
<script>
function fetchGet () {
// (A) DATA TO SEND
var data = {
name : "jon doe",
email : "jon@doe.com"
};
// (B) MANUAL CONSTRUCT URL & PARAMS
var url = "http://site.com/0-dummy.php";
url += "?";
for (let k in data) { url += k + "=" + data[k] + "&"; }
url = encodeURI(url.slice(0, -1));
// (C) FETCH WITH GET SEARCH PARAMS
fetch(url)
// (D) RETURN SERVER RESPONSE AS TEXT
.then(res => {
console.log(res);
if (res.status != 200) { throw new Error("Bad Server Response"); }
return res.text();
})
.then(res => console.log(response))
// (E) HANDLE ERRORS (OPTIONAL)
.catch(err => console.error(err));
}
</script>
<input type="button" value="Fetch Get" onclick="fetchGet()">
If you have to support older browsers, the only way is to manually construct the URL string and parameters.
- Simply append the parameters behind the URL –
http://site.com/path?KEY=VALUE&KEY=VALUE
- Then encode the URL using
encodeURI()
.
DOWNLOAD & NOTES
Here is the download link to the example code, so you don’t have to copy-paste everything.
SORRY FOR THE ADS...
But someone has to pay the bills, and sponsors are paying for it. I insist on not turning Code Boxx into a "paid scripts" business, and I don't "block people with Adblock". Every little bit of support helps.
Buy Me A Coffee Code Boxx eBooks
EXAMPLE CODE DOWNLOAD
Click here for the source code on GitHub gist, just click on “download zip” or do a git clone. I have released it under the MIT license, so feel free to build on top of it or use it in your own project.
EXTRA BITS & LINKS
That’s all for the tutorial, and here is a small section on some extras and links that may be useful to you.
COMPATIBILITY CHECKS
- Arrow Functions – CanIUse
- Fetch API – CanIUse
- URL Search Params – CanIUse
The examples will work on all modern browsers. Old Internet Exploders, beware.
LINKS & REFERENCES
- Fetch API – MDN
- POST Form Data With Fetch – Code Boxx
INFOGRAPHIC CHEAT SHEET
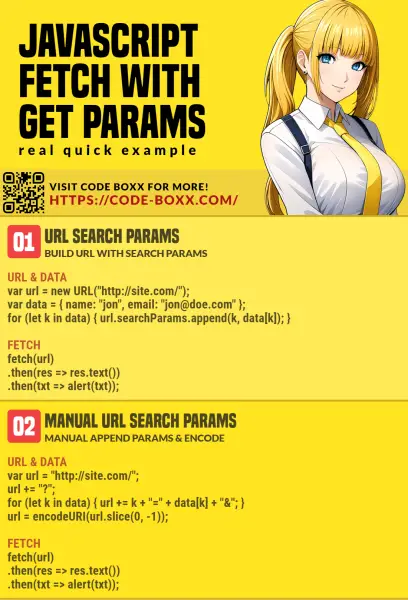
THE END
Thank you for reading, and we have come to the end. I hope that it has helped you to better understand, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!