Welcome to a quick beginner’s tutorial on how to reset an HTML form after submission. So you are working on a form that needs to be cleared after the user submits it?
There are 2 mechanisms in HTML and Javascript to take note of when it comes to resetting forms:
- Use
document.getElementById("FORM").reset()
in Javascript to reset the form. - Add an
autocomplete="off"
property to the HTML form to prevent possible auto-filling after the form reset.
That covers the basics, but let us walk through a few examples in this guide – Read on!
TLDR – QUICK SLIDES
[web_stories_embed url=”https://code-boxx.com/web-stories/reset-html-form-after-submission/” title=”Reset HTML Form After Submission” poster=”https://code-boxx.com/wp-content/uploads/2021/11/STORY-JS-20230518.webp” width=”360″ height=”600″ align=”center”]
Fullscreen Mode – Click Here
TABLE OF CONTENTS
FORM RESET
All right, let us now get into the examples of resetting the form after submission.
EXAMPLE 1) AUTOCOMPLETE OFF
<form method="post" autocomplete="off">
Name: <input type="text" name="user-name" required>
Email: <input type="email" name="user-email" required>
<input type="submit" value="Go!">
</form>
First, this is nothing but a simple HTML form with autocomplete="off"
. What has this got to do with resetting forms? Heard of “autocomplete” or “autofill”? This modern convenience can be a stupid pain when resetting forms – You reset the form, and autocomplete fills them back. Genius.
So yes, autocomplete="off"
is optional, but recommended. Take note though, this is not a directive but more of a recommendation. Browsers and plugins can still ignore and do the form autocomplete.
EXAMPLE 2) AJAX FORM SUBMIT & RESET
2A) HTML FORM
<form id="my-form" autocomplete="off" onsubmit="return process()">
<!-- (A) TAKE NOTE: THIS WILL RESET TO "JOHN DOE", NOT EMPTY. -->
Name: <input type="text" name="name" value="John Doe" required>
<!-- (B) THIS WILL REVERT TO BLANK -->
Email: <input type="email" name="email" required>
<input type="submit" value="Go!">
</form>
In this example, we have an HTML form that submits via AJAX. There is nothing special here, take note of the value
though:
<input type="text" id="user-name" value="John Doe">
This field will reset to “John Doe”, not blank.<input type="email" id="user-email">
This will reset to a blank field.
2B) THE JAVASCRIPT
function process () {
// (A) GET FORM DATA
var form = document.getElementById("my-form"),
data = new FormData(form);
// (B) AJAX SEND FORM
// NOTE: USE HTTP://. NOT FILE://.
fetch("x-dummy.txt", { method: "POST", body: data })
.then(res => res.text())
.then(txt => {
console.log(txt);
form.reset(); // reset form
});
// (C) STOP DEFAULT FORM SUBMIT/PAGE RELOAD
return false;
}
This may throw the absolute beginners off a little bit because we are not submitting the form in the “traditional way”. This is called Asynchronous Javascript And XML (AJAX), in short, submitting the form without reloading the page. Now, it may seem to be a small handful at first, but it is very straightforward.
- First, get the HTML form
var form = document.getElementById("my-form")
. Then, collect the form datadata = new FormData(form)
. - We use
fetch(URL, { method: "POST", body: data })
to send the form to the server via an AJAX call..then(res => res.text())
Returns the server response as plain text..then(txt => { DO SOMETHING })
Do something with the server response. In this simple example, we useform.reset()
to reset the form.
There, it’s not really that difficult.
EXAMPLE 3) PARTIAL FORM RESET
3A) HTML FORM
<form id="my-form" autocomplete="off" onsubmit="return process()">
Email: <input type="email" name="email" required>
Password: <input type="password" id="user-password" name="password" required>
Confirm Password: <input type="password" id="confirm-password" name="cpassword" required>
<input type="submit" value="Go!">
</form>
A dummy HTML form as usual, but this time with 3 fields – Email, password, and confirm password. After submitting, we will reset the password fields, but leave the email be.
3B) THE JAVASCRIPT
function process () {
// (A) GET FORM DATA
var data = new FormData(document.getElementById("my-form"));
// (B) AJAX SEND FORM
fetch("x-dummy.txt", { method: "POST", body: data })
.then(res => res.text())
.then(txt => {
console.log(txt);
document.getElementById("user-password").value = ""; // reset password
document.getElementById("confirm-password").value = ""; // reset confirm password
});
// (C) STOP DEFAULT FORM SUBMIT/PAGE RELOAD
return false;
}
This is pretty much the same old AJAX process. But to partially reset a form, there is sadly no quick way around it. We have to manually get the fields and reset their values one by one.
DOWNLOAD & NOTES
Here is the download link to the example code, so you don’t have to copy-paste everything.
SORRY FOR THE ADS...
But someone has to pay the bills, and sponsors are paying for it. I insist on not turning Code Boxx into a "paid scripts" business, and I don't "block people with Adblock". Every little bit of support helps.
Buy Me A Coffee Code Boxx eBooks
EXAMPLE CODE DOWNLOAD
Click here for the source code on GitHub gist, just click on “download zip” or do a git clone. I have released it under the MIT license, so feel free to build on top of it or use it in your own project.
EXTRA BITS & LINKS
That’s all for the tutorial, and here is a small section on some extras and links that may be useful to you.
LINKS & RECOMMENDED READS
- Javascript AJAX – A Beginner’s Tutorial – Code Boxx
- HTML Form Validation – Code Boxx
- HTML Autocomplete attribute – MDN
INFOGRAPHIC CHEAT SHEET
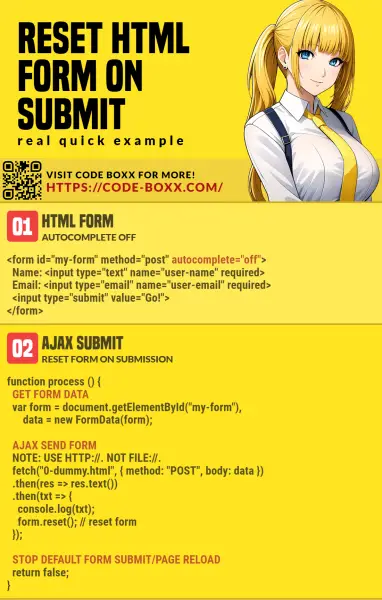
THE END
Thank you for reading, and we have come to the end of this guide. I hope that it has helped you with your project, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!
I’m very new to AJAX and JS in general, so I guess I’m missing something obvious: Most of your code is clear and understandable, but I don’t see where you specify the HTTP server and the name of the program that is supposed to recieve this data on the other end. Also, does it arrive as POST, or GET, or something else? For example, if I wanted a PHP program to receive the data on the server, how would I do that?
Thanks!
???????????????????????????
POST –
fetch("URL", { method: "POST", body: data })
GET –
fetch("URL?KEY=VALUE&KEY=VALUE")
https://code-boxx.com/javascript-ajax-beginners/