Welcome to a beginner’s tutorial on Javascript loops and iterations. Want to loop through arrays and objects, or do things repetitively? The common mechanisms to loop and iterate in Javascript are:
For | for (let i=0; i<10; i++) { ... } |
For-In | for (let KEY in OBJECT/ARRAY/STRING) { ... } |
For-Of | for (let VALUE of ARRAY/STRING) { ... } |
Foreach | ARRAY.foreach((VALUE, KEY) => { ... }); |
For-Object entries | for (let [KEY, VALUE] of Object.entries(OBJECT)) { ... } |
While | while (i<10) { i++; } |
Do-While | do { i++; } while (i<10); |
Break | while (i<10) { if (i<0) { break; } i++; } |
Continue | while (i<10) { if (i==2) { i+=2; continue; } i++; } |
That covers the quick basics at a glance, but let us walk through examples of each – Read on!
TLDR – QUICK SLIDES
[web_stories_embed url=”https://code-boxx.com/web-stories/javascript-loops-iterations/” title=”Javascript Loops & Iterations” poster=”https://code-boxx.com/wp-content/uploads/2021/11/STORY-JS-20230518.webp” width=”360″ height=”600″ align=”center”]
Fullscreen Mode – Click Here
TABLE OF CONTENTS
JAVASCRIPT LOOPS & ITERATIONS
All right, let us now get into the examples of loops and iterations in Javascript.
1) FOR
// (A) INCREMENT
for (let i=1; i<=3; i++) {
console.log(i); // 1, 2, 3
}
// (B) DECREMENT
for (let i=3; i>=1; i--) {
console.log(i); // 3, 2, 1
}
// (C) LOOP ARRAY
var arr = ["a", "b", "c"];
for (let i=0; i<arr.length; i++) {
console.log([i]); // 0, 1, 2
console.log(arr[i]); // a, b, c
}
// (D) WE CAN ACTUALLY OMIT ALL INIT & CONDITIONS
var i = 0;
for (;;) {
console.log(i); // 0, 1
i++;
if (i==2) { break; }
}
The for
loop is one of the most commonly used, and some books don’t even explain it right. Take note that there are “3 segments” – for (INITIALIZE; END CONDITION; STEP)
.
INITIALIZE
This runs before the loop starts.END CONDITION
The condition to stop looping, this statement must resolve to a booleantrue/false
. Runs afterINITIALIZE
and after every cycle.STEP
Run this after every cycle.
Yes, we can leave all 3 empty… But that will not make any sense.
2) FOR-IN
// (A) ITERATE OBJECT
var person = {
name : "Jon Doe",
email : "jon@doe.com"
};
for (let key in person) {
console.log(key); // name, email
console.log(person[key]); // jon, jon@doe.com
}
// (B) ITERATE ARRAY
var animals = ["Birb", "Cate", "Doge"];
for (let i in animals) {
console.log(i); // 0, 1, 2
console.log(animals[i]); // birb, cate, doge
}
// (C) ITERATE STRING
var hello = "world";
for (let i in hello) {
console.log(i); // 0, 1, 2, 3, 4
}
The for-in
loop will run through the keys:
- Properties of an object.
- The index of arrays.
- Character position of a string.
3) FOR-OF
/* (A) ITERATE OBJECT - ERROR, NOT ITERABLE
var obj = { "foo" : "bar" };
for (let value of obj) {
console.log(value);
}*/
// (B) ITERATE ARRAY
var animals = ["Birb", "Cate", "Doge"];
for (let value of animals) {
console.log(value); // birb, cate, doge
}
// (C) ITERATE STRING
var hello = "world";
for (let char of hello) {
console.log(char); // w, o, r, l, d
}
This should be pretty self-explanatory by now, for-in
iterates the properties, for-of
iterates the values (data) themselves.
4) FOREACH
var animals = ["Birb", "Cate", "Doge"];
animals.forEach((value, key) => {
console.log(value); // birb, cate, doge
console.log(key); // 0, 1, 2
});
The forEach()
function only applies to arrays – It loops through all the elements within the array.
5) FOR & OBJECT ENTRIES
// (A) PERSON OBJECT
var person = {
"Name" : "Jon",
"Email" : "jon@doe.com"
};
// (B) LOOP OBJECT ENTRIES
for (let [key, value] of Object.entries(person)) {
console.log(key); // name, email
console.log(value); // jon, jon@doe.com
}
This is the “smart and convenient” way to loop through both the key and values of an object within a single loop.
6) WHILE
var animals = ["Birb", "Cate", "Doge"], i = 0;
while (i < animals.length) {
console.log(i); // 0, 1, 2
console.log(animals[i]); // birb, cate, doge
i++;
}
A while
loop is pretty simple – It will continue to run until the provided condition (i < animals.length
) turns false… But beware, a common mistake that many newbies make is an end condition that will never be met, ending up in an infinite loop.
7) DO-WHILE
var animals = ["Birb", "Cate", "Doge"], i = 0;
do {
console.log(i); // 0, 1, 2
console.log(animals[i]); // birb, cate, doge
i++;
} while (i < animals.length);
Look no further, this does almost the exact same thing as while
. What is the difference here?
while
will not even start if the condition is not met.do-while
will run at least once, even when the condition is not met.
8) BREAK & CONTINUE
let i = 0;
while (i>=0) {
// (A) CONTINUE - SKIP TO NEXT CYCLE
if (i==2) {
i+=2;
continue;
}
i++;
console.log(i); // 1, 2, 5, 6
// (B) BREAK - STOP CYCLE
if (i==6) { break; }
}
Lastly, we have another 2 Captain Obvious mechanisms:
continue
Skip the current cycle.break
Stop the entire loop. Works infor for-in for-of foreach
too.
DOWNLOAD & NOTES
Here is the download link to the example code, so you don’t have to copy-paste everything.
SORRY FOR THE ADS...
But someone has to pay the bills, and sponsors are paying for it. I insist on not turning Code Boxx into a "paid scripts" business, and I don't "block people with Adblock". Every little bit of support helps.
Buy Me A Coffee Code Boxx eBooks
EXAMPLE CODE DOWNLOAD
Click here for the source code on GitHub gist, just click on “download zip” or do a git clone. I have released it under the MIT license, so feel free to build on top of it or use it in your own project.
EXTRA BITS & LINKS
That’s all for the tutorial, and here is a small section on some extras and links that may be useful to you.
LINKS & REFERENCES
- For loop – MDN
- While loop – MDN
- For-in – MDN
- For-of – MDN
- Break – MDN
- Continue – MDN
- Object Entries – MDN
INFOGRAPHIC CHEAT SHEET
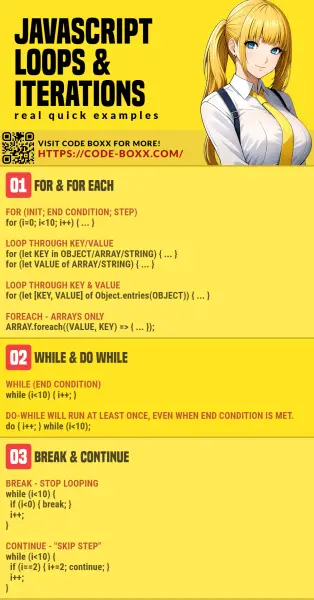
THE END
Thank you for reading, and we have come to the end. I hope that it has helped you to better understand, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!